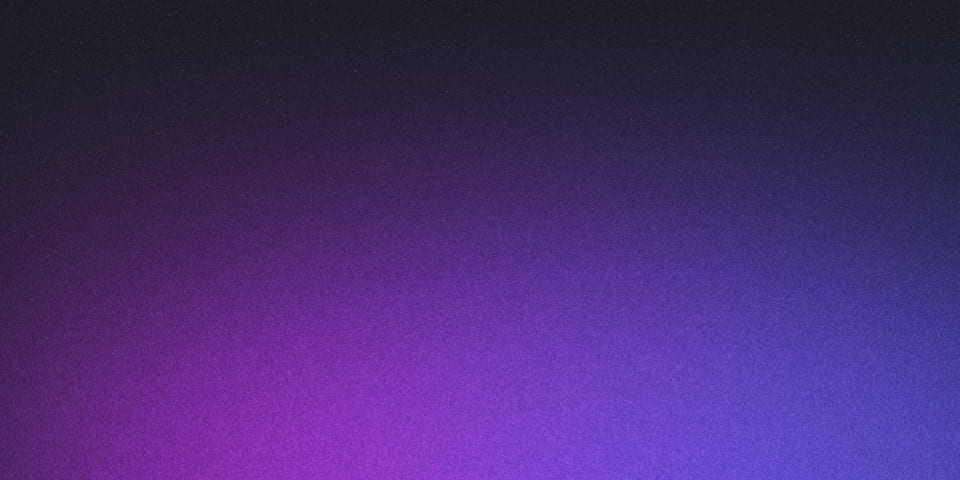
Should you test ORM code on a real database?
tl;dr - yes
So…
I like Entity Framework.
This may be a controversial view. But I like it.
I like that I can just write C# and get a sane database schema and a sane query produced.
What I dislike is when we don’t consider the impact of not testing the ORM driver early.
Let’s take the following example which insertion of an object into a context using the Microsoft.EntityFrameworkCore.Sqlite
driver (and just running in a test so I can get easy pretty visuals).
Sqlite makes sense for tests, light weight, cheap, disposable, love it.
[Fact]
public async Task TestSqlite() {
var context = new SqliteContext();
await context.Entries.AddAsync(new Entry {
DateField = DateTime.Now
});
await context.SaveChangesAsync()
}
That works.
Should it? Does that give me any confidence that the code I just wrote is actually going to run in production?
Let’s flip this, let’s run on Postgres using TestContainers to start a database instance that’s isolated to this test.
[Fact]
public async Task PostgresTest()
{
var pgContainerBuilder = new PostgreSqlBuilder().Build();
await pgContainerBuilder.StartAsync();
await using var context = new PostgresContext(pgContainerBuilder.GetConnectionString());
await context.Database.EnsureCreatedAsync();
await context.Entries.AddAsync(new Entry { DateField = DateTime.Now });
await context.SaveChangesAsync();
}
We are doing the same code against the EF context, right?
The same insert operation works the same way, right?
System.ArgumentException
Cannot write DateTime with Kind=Local to PostgreSQL type 'timestamp with time zone', only UTC is supported. Note that it's not possible to mix DateTimes with different Kinds in an array, range, or multirange. (Parameter 'value')
That…
Oh…
While it’s nice to use SQLite for the sake of quick/fast validation, I can easily fall into scenarios where I haven’t validated my code actually makes sense for how it’s going to run.
Testing my ORM logic against SQL Server when I’m running Postgres makes no sense and testing an abstraction that relies on DB specific implementation details against literally any other database leads to false confidence at best and incidents caused by belief your tests are correct at the worst.
To clarify for the sake of saying this, I do really really love SQLite as a tool for quick/iterative development, was just a known example that I could use to illustrate this point! Use the right tech at the right time. Testing on a database you do not use is not the right tech.